How to find element by text using Selenium
Important: we’ll use a real-life example in this tutorial, so you’ll need the Selenium library and browser drivers installed.
from selenium.webdriver.common.by import By
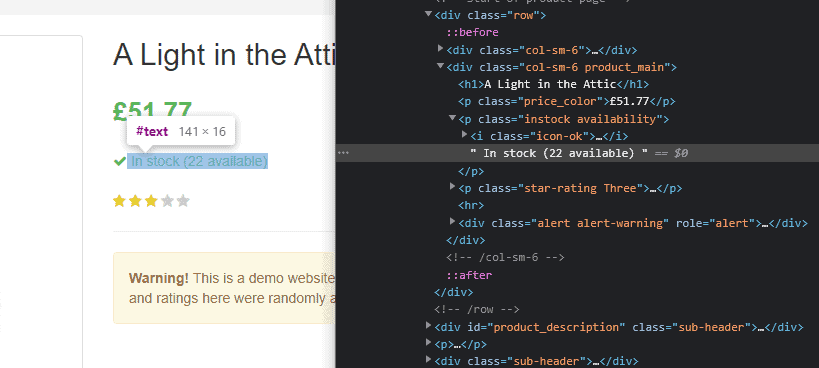
//*[contains (text(),'stock')]
It finds any element within the page, the text of which contains a stock string.
element_by_text = driver.find_element(By.XPATH, "//*[contains (text(),'stock')]").text
print (element_by_text)
NOTE: We’re using the driver.find_element() function to only get the first element found by the selector. It’s also possible to use the driver.find_elements() function to get a list of all elements.
This is the output of the script. It shows the elements you’ve just scraped.

Step 5. Now we can clean up the result by extracting the number from the text to do some other operations with it as an integer variable. You can do that with some simple regex.
Here we find the decimal number within the element_by_text string, assign it to a new variable and print it out separately:
in_stock = re.findall(r'\d+', element_by_text)[0]
print (f'In stock: {in_stock}')
This is the output of the script. It shows the stock availability of a book you’ve just scraped.

Results:
Congratulations, you’ve just extracted the stock availability of a book using Selenium.
from selenium import webdriver
from selenium.webdriver.common.by import By
import re
driver = webdriver.Chrome()
driver.get("http://books.toscrape.com/catalogue/a-light-in-the-attic_1000/index.html")
element_by_text = driver.find_element(By.XPATH, "//*[contains (text(),'stock')]").text
driver.quit()
print (element_by_text)
in_stock = re.findall(r'\d+', element_by_text)[0]
print (f'In stock: {in_stock}')