How to Scrape Google Search Results
We explore what tools are the best for scraping Google search results.
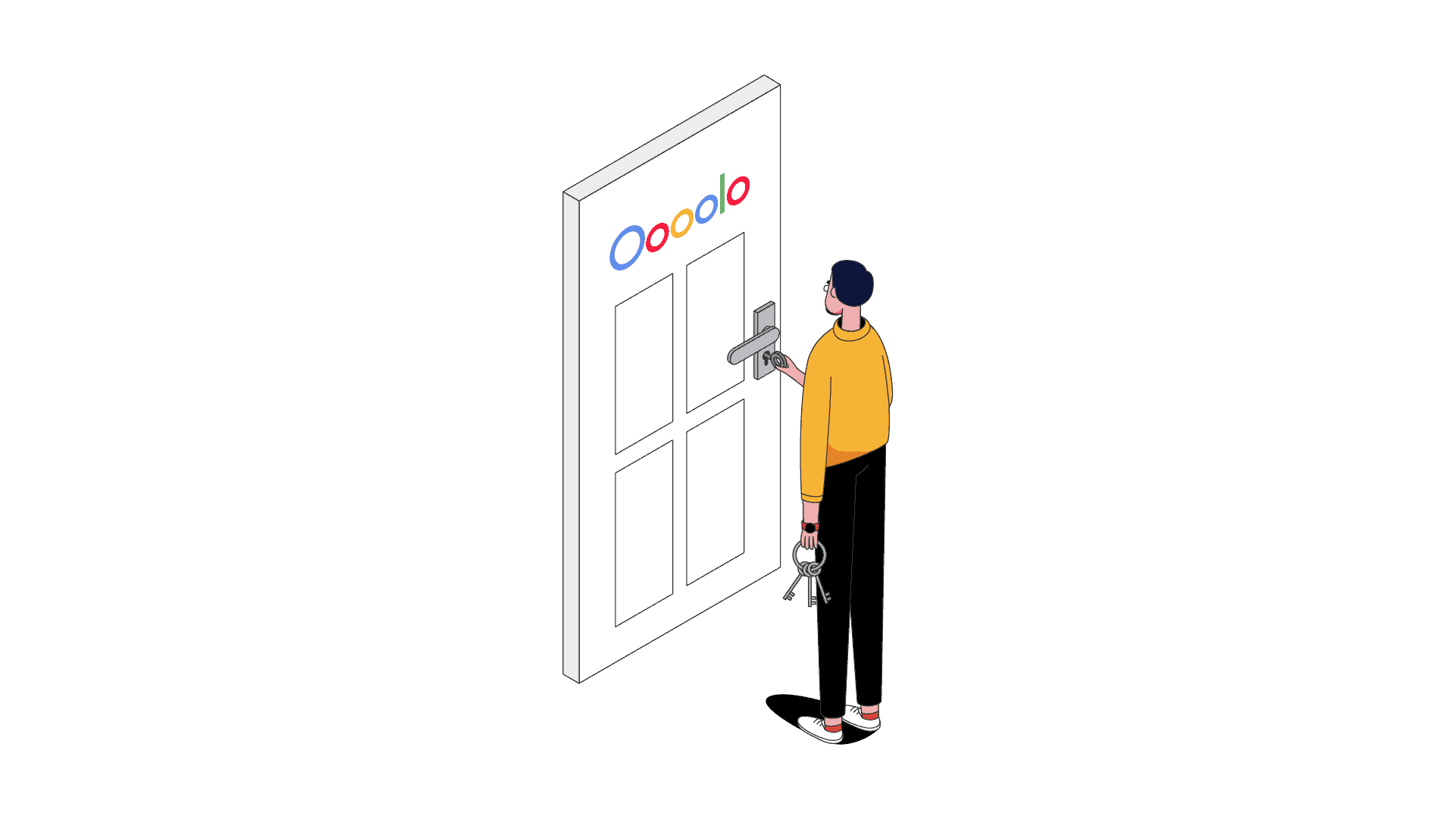
As of January 2025, Google has started requiring JavaScript rendering to load.
Google recently launched an update requiring JavaScript rendering for displaying Google search results. Without it, Google refuses to deliver the query and rather redirects to instructions for enabling JavaScript. As of now, the developer community is still looking for solutions of how to easily build scrapers for search results.
Therefore, you’re looking to scrape Google search results, your best bet is using a SERP API – a dedicated tool made specifically for extracting search queries.
Find the best SERP API tools currently available on the market.
Wait – What About the Google Search API?
- The API is made for searching within one website or a small group of sites. You can configure it to search the whole web but that requires tinkering.
- The API provides less and more limited information compared to both the visual interface and web scraping tools.
- The API costs a lot of money: 1,000 requests will leave you $5 poorer, which is a daylight robbery. There are further limits on the number of daily requests you can make.
Overall, the Google Search API isn’t really something you’d want to use considering its limitations. Trust me, you’ll save both money and sanity by going the web scraping route.
Are there any other ways to scrape Google Search apart from a SERP API? Yes. The alternatives would be to use a visual web scraper, browser extension, or data collection service. Let’s briefly run through each.
Visual Web Scraper
Visual web scrapers are programs that let you extract data from Google without any coding experience. They give you a browser window, where you simply point and click the data points you want to scrape and download them in the format of your choice. The hardest part is building a proper workflow of paginations and action loops, but that’s still easy compared to writing the code by yourself.
When should you get a visual web scraper? When you need a small-moderate amount of data and have no coding experience.
Which visual web scrapers to use? ParseHub and Octoparse are two great options. We’re partial to Octoparse because it has a lighter UI and premade templates for quick basic scraping.
Data Collection Service
A data collection service is the easiest method for getting data from Google Search. You specify your requirements, budget, and then receive the results all nicely formatted for further use. That’s about it. You don’t need to build or maintain a scraper, worry about the scraping logic, or even the legal aspects of your scraping project. Your only worry will be money.
When should you use a data collection service? This one’s pretty simple: when you’re running a mid to large-scale project, have the funds, and no one to build a web scraper for you.
Which data collection service to choose? There’s no shortage of companies that provide data collection services. Some examples would be ScrapingHub and Bright Data.
Google Search Results Dataset
If your project allows, you can choose to get a dataset instead of collecting SERP data yourself. Some dataset services, like Kaggle, have a collection of SERP datasets ready for analysis.
Here, however, it’s important to remember that SERP data changes often, and datasets likely won’t be refreshed as often. So, this would be a viable option if you’re interested in historical data.

Frequently Asked Questions About How to Scrape Google Search Results
Google doesn’t like being scraped – and has mechanisms to limit it – but we haven’t heard about it suing anyone over scraping Google Search. If it did, many SEO companies would simply go out of business.
Yes, there is. But it’s limited and expensive (1,000 queries cost $5), so people turn to web scraping instead.
Take a look at our list of the best proxies for Google scraping.